B
2020
Theme Development
Website Refresh | Custom WordPress Theme
Client: Siobhan Wolff Photography
URL: siobhanwolff.photography
Designs: Hayley Robinson
Web Development: Bartosz
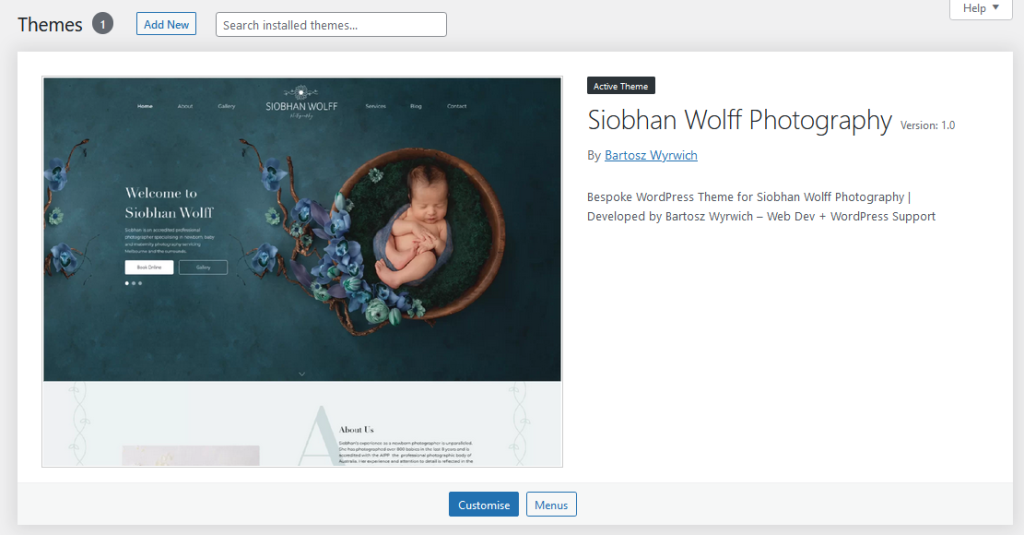
Fast, lightweight and responsive
This custom PHP development centres on a bespoke lightweight, minimalist WordPress theme that utilises Gutenberg block editor .
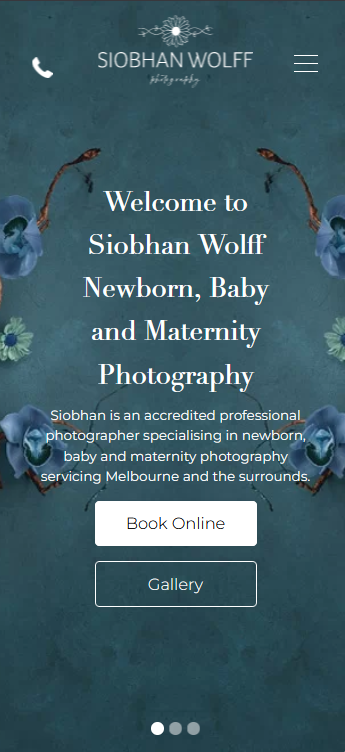
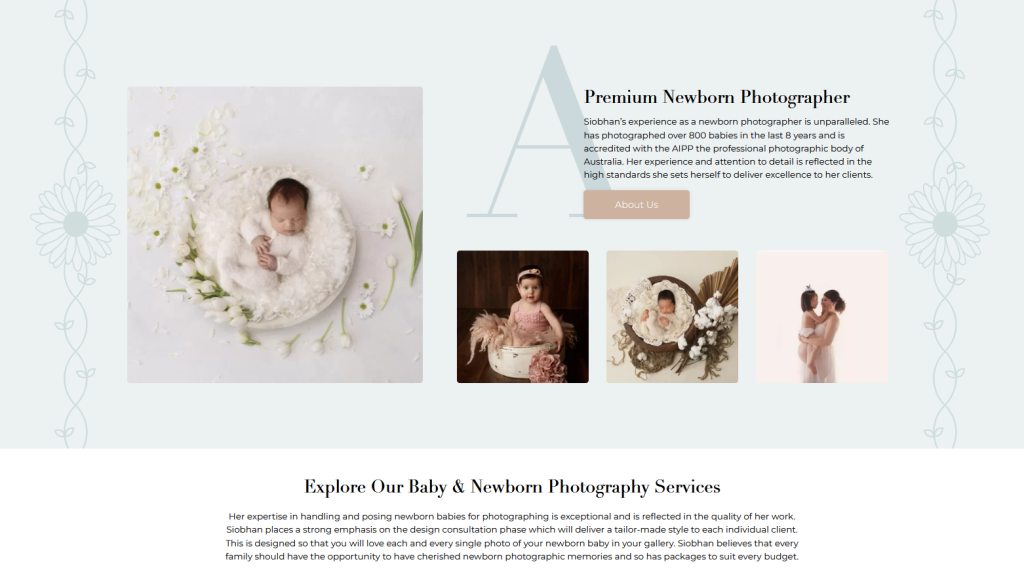
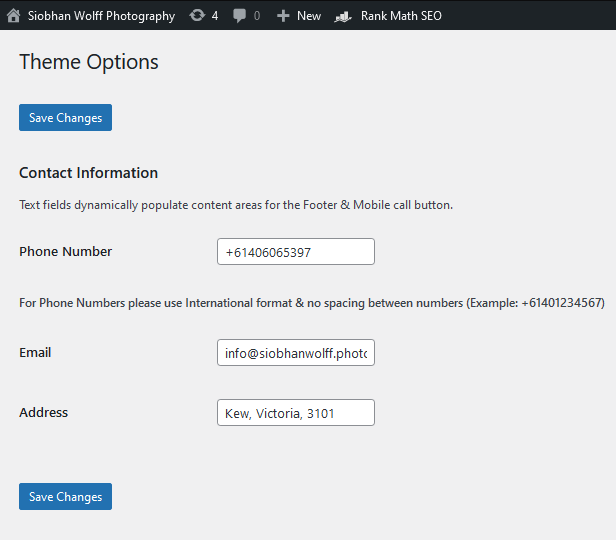
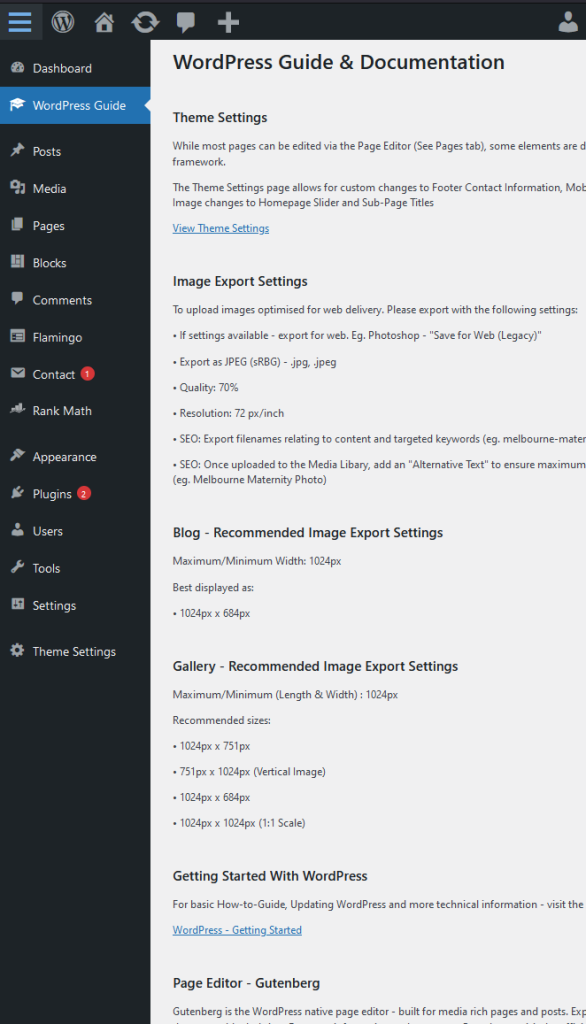
// Excerpt from functions.php
function siobhan_setup() {
add_theme_support( 'title-tag' );
add_theme_support( 'post-thumbnails' );
add_theme_support( 'automatic-feed-links' );
}
add_action( 'after_setup_theme', 'siobhan_setup' );
// Adding custom image sizes
if ( function_exists( 'add_theme_support' ) ) {
add_image_size( 'entry-title-image', 1311, 616 );
add_image_size( 'first-archive-thumb', 864.5, 500.71 );
add_image_size( 'archive-thumb', 641, 428 );
add_image_size( 'featured-main', 417.5, 278.8 );
add_image_size( 'blog-thumb-cut', 417, 235 );
}
// Proper enqueue of scripts - including GoogleFonts, AdobeTypeKit, and Custom CSS & JSS
function siobhan_script_enqueue() {
wp_enqueue_style( 'google-fonts', '//fonts.googleapis.com/css2?family=Montserrat:wght@300;400;500;600&display=swap', false );
wp_enqueue_style('adobe-typekit', '//use.typekit.net/skl0srx.css', false );
wp_enqueue_style('siobhanstyle', get_template_directory_uri() . '/css/siobhan.css', array(), false, 'all');
wp_register_script( 'siobhanscript', get_template_directory_uri() . '/js/siobhan.js', array(), false, true );
wp_enqueue_script( 'siobhanscript' );
}
add_action( 'wp_enqueue_scripts','siobhan_script_enqueue');
// Optimisation: Using JQuery from a faster loading CDN hosted by googleapis.com
* Removes jQuery that comes with WordPress. Loads custom jQuery version - and adds cross-origin & integrity
* original: //code.jquery.com/jquery-3.5.1.min.js
*
* Deregister included jQuery
* Add custom jQuery with Crossorigin + Integrity
*/
function include_custom_jquery() {
wp_deregister_script('jquery');
wp_enqueue_script('jquery', 'https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js', array(), null, true);
wp_deregister_script( 'jquery-migrate' );
wp_enqueue_script('jquery-migrate', '//code.jquery.com/jquery-migrate-3.3.0.min.js', array('jquery'), '3.3.0', false );
}
// Add integrity and cross origin attributes to the jQuery script.
function add_custom_jquery_script_attributes( $html, $handle ) {
if ( $handle === 'jquery' ) {
return str_replace( '></script>', ' integrity="sha256-9/xXx1Xx1x1XXx1x1/xXX1Xx1xX1xx1xX1x1x=" crossorigin="anonymous"></script>', $html );
}
return $html;
}
add_filter('script_loader_tag', 'add_custom_jquery_script_attributes', 10, 2);
// Add integrity and cross origin attributes to the jQuery migrate script.
function add_custom_jquerymigrate_script_attributes( $html, $handle ) {
if ( $handle === 'jquery-migrate' ) {
return str_replace( '></script>', ' integrity="sha256-xXX1Xx1xX1xx1xX1x1x1Xx1x1XXx1x1=" crossorigin="anonymous"></script>', $html );
}
return $html;
}
add_filter('script_loader_tag', 'add_custom_jquerymigrate_script_attributes', 10, 2);
add_action('wp_enqueue_scripts', 'include_custom_jquery');
// Adding Bootstrap & dependencies
/**
* Load bootstrap from CDN
* https://getbootstrap.com/
*/
function enqueue_load_bootstrap() {
// Add bootstrap js
wp_register_script( 'bootstrap-js', 'https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.5.0/js/bootstrap.min.js', ['jquery'], NULL, true );
wp_enqueue_script( 'bootstrap-js' );
// Add bootstrap CSS
wp_register_style( 'bootstrap-css', 'https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.5.0/css/bootstrap.min.css', false, NULL, 'all' );
wp_enqueue_style( 'bootstrap-css' );
// Add popper js
wp_register_script( 'popper-js', 'https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.16.0/umd/popper.min.js', ['jquery'], NULL, true );
wp_enqueue_script( 'popper-js' );
}
// Adding Bootstrap JS integrity and cross origin attributes
function add_bootstrap_script_attributes( $html, $handle ) {
if ( $handle === 'bootstrap-js' ) {
return str_replace( '></script>', ' integrity="sha384-xXX1Xx1xx11x1Xx1x1XXx1x1+xXX1Xx1xX1xx1x1XXx1x1" crossorigin="anonymous"></script>', $html );
}
return $html;
}
add_filter('script_loader_tag', 'add_bootstrap_script_attributes', 10, 2);
// Adding Bootstrap CSS integrity and cross origin attributes
function add_bootstrap_css_attributes( $html, $handle ) {
if ( $handle === 'bootstrap-css' ) {
return str_replace( '/>', 'integrity="sha384-xXX1Xx1xX1xx1xX1x1Xx1x1+xXX1Xx1xX" crossorigin="anonymous" />', $html );
}
return $html;
}
add_filter( 'style_loader_tag', 'add_bootstrap_css_attributes', 10, 2 );
// Adding Bootstrap Popper JS integrity and cross origin attributes
function add_popper_script_attributes( $html, $handle ) {
if ( $handle === 'popper-js' ) {
return str_replace( '></script>', ' integrity="sha384-xXX1Xx1xX1xx1x+xXX1Xx1xX1xx1xX1x1x1Xx1x1XXx1x1xXX1Xx1xX1xx1xX1x1x1Xx1x1XXx1x1" crossorigin="anonymous"></script>', $html );
}
return $html;
}
add_filter('script_loader_tag', 'add_popper_script_attributes', 10, 2);
add_action( 'wp_enqueue_scripts', 'enqueue_load_bootstrap' );
// Optimisation: Remove dashicons unless admin logged in
function bs_dequeue_dashicons() {
if ( ! is_user_logged_in() ) {
wp_deregister_style( 'dashicons' );
}
}
add_action( 'wp_enqueue_scripts', 'bs_dequeue_dashicons' );
// Add Bootstrap classes to WordPress menu
function li_class_navitem($classes, $item, $args) {
$classes[] = 'nav-item';
return $classes;
}
add_filter('nav_menu_css_class','li_class_navitem',1,3);
function my_deregister_scripts(){
wp_deregister_script( 'wp-embed' );
}
add_action( 'wp_footer', 'my_deregister_scripts' );
// Add second menu for left and right desktop versions
function main_menu_split() {
register_nav_menus(
array(
'menu-left' => __( 'menu-left' ),
'menu-right' => __( 'menu-right' )
)
);
}
add_action( 'init', 'main_menu_split' );
// Post excerpt - change character length
function new_excerpt_length($length) {
return 45;
}
add_filter('excerpt_length', 'new_excerpt_length');
/// Post excerpt - add '...' to the end of excerpt
function new_excerpt_more($more) {
return '...';
}
add_filter('excerpt_more', 'new_excerpt_more');
Highlights
This website build maximises the use of the WordPress framework with a minimalist code approach to custom theme development with efficient and accessible code practises in mind.
Custom PHP Theme with Bootstrap CSS, and content using Gutenberg blocks.
PHP page template development – custom blog archive and pages matching design concepts.
Custom admin panels: theme documentation and theme settings including quick editable fields for contact information.
// Use of Theme Options input fields
<?php
// Phone Number
$foonum = siobhan_get_theme_option( 'phonenumber' );
// Converting international phone format for button label
$str = substr($foonum, 3);
$foonumF = "0" .chunk_split($str, 3, ' ');
// Email and Address
$footeremail = siobhan_get_theme_option( 'emailadd' );
$footeraddy = siobhan_get_theme_option( 'addyadd' );
?>
// Call Button
<a alt="Call Now" href="tel:<?php echo $foonum;?>">
<?php echo $foonumF;?>
</a>
<?php
/*
* Template Name: Bump to Baby
* description: >-
Page template for a service sub-page with unique design features.
*/
get_header(); ?>
<div class="mainbg"> </div>
<div id="bumptobaby">
// Adding the WordPress loop
<?php if (have_posts()): while (have_posts()) : the_post(); ?>
// Theme Options - Feature image switches to different image URLs based on media sizes. Allows editors to adjust photography for optimal responsive display.
<?php
$fisbbd = siobhan_get_theme_option( 'services_bumptobaby_desktop' );
$fisbbt = siobhan_get_theme_option( 'services_bumptobaby_tablet' );
$fisbbm = siobhan_get_theme_option( 'services_bumptobaby_mobile' );
?>
<div class="entry-container container p-md-0 z-5">
<div class="wolff-flower">
<div class="wp-block-image">
<figure class="aligncenter size-full"><img src="/wp-content/uploads/2020/05/wolff_flower.svg" alt="Siobhan Wolff Photography" class="wp-image-247"></figure>
</div>
</div>
<div class="entry-image ei-desktop" style="background-image: url('<?php echo $fisbbd; ?>');">
<div class="entry-title">
<a href=" <?php the_permalink(); ?>"><h1><?php the_title(); ?></h1>
</a>
<a href="/contact/"> <button class="lightbtn">Contact Us</button>
</a>
</div>
</div>
<div class="entry-image ei-tablet" style="background-image: url('<?php echo $fisbbt; ?>');">
<div class="entry-title">
<a href=" <?php the_permalink(); ?>"><h1><?php the_title(); ?></h1>
</a>
</div>
</div>
<div class="entry-image ei-mobile" style="background-image: url('<?php echo $fisbbm; ?>');">
<div class="entry-title">
<a href=" <?php the_permalink(); ?>"><h1><?php the_title(); ?></h1>
</a>
</div>
</div>
</div>
// Visual elements displayed on >large desktop
<div class="side-flower-left">
<div class="wp-block-image">
<figure class="aligncenter size-full"><img src="/wp-content/uploads/2020/05/wolff_flower.svg" alt="Newborn Photography" class="wp-image-247"></figure>
</div>
</div>
<div class="side-flower-right">
<div class="wp-block-image">
<figure class="aligncenter size-full"><img src="/wp-content/uploads/2020/05/wolff_flower.svg" alt="Baby Photography" class="wp-image-247"></figure>
</div>
</div>
// Load in page content
<div class="content z-10 position-relative">
<?php the_content(); ?>
</div>
<?php endwhile; ?>
<?php endif; ?>
</div>
// Footer
<?php get_footer(); ?>